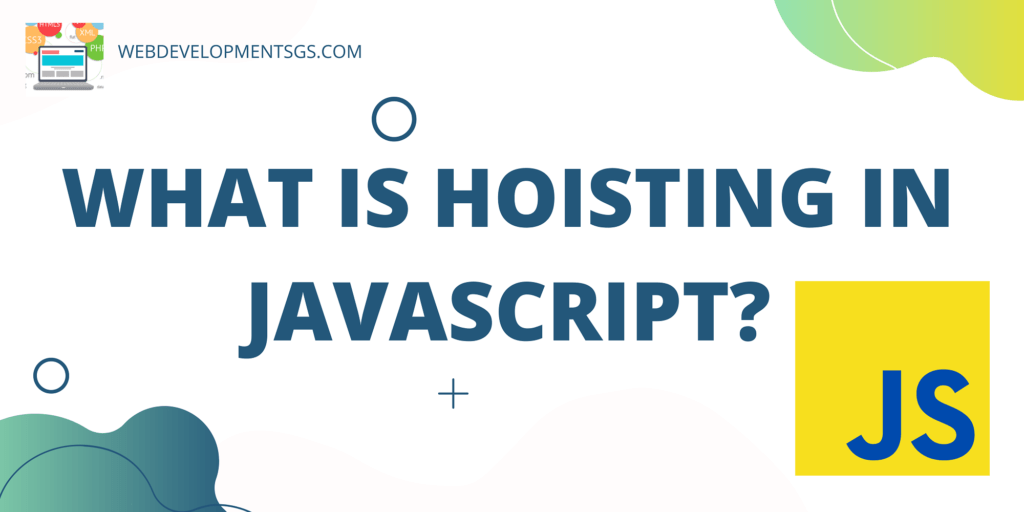
Welcome to our comprehensive guide on hoisting in JavaScript. In this article, we’ll dive deep into the concept of hoisting, exploring its intricacies, and uncovering its full potential.
Table of Contents
- What is Hoisting in Javascript?
- How the Hoisting Mechanism works
- Hoisting with var
- Hoisting with let and const
- Function Hoisting
- Block Scoping and Hoisting
- Benefits of Hoisting in Javascript
- Hoisting Pitfalls
- Hoisting Best Practices
1. What is Hoisting in Javascript?
In Javascript Hoisting is a mechanism where variables and function declarations are moved to the top of their containing scope during the compile phase, before the code execution.
2. Hoisting Mechanism
The hoisting mechanism involves two steps:
- Variable declarations are hoisted, but not their initializations.
- Function declarations are fully hoisted, including their function bodies.
3. Hoisting with var
Variables declared with var are hoisted to the top of their scope. However, only the declaration is hoisted, not the initialization.
console.log(x); // Output: undefined
var x = 10;
4. Hoisting with let and const
Variables declared with let and const are hoisted, but they are not initialized until their actual declaration is evaluated.
console.log(y); // Throws ReferenceError: Cannot access 'y' before initialization
let y = 20;
5. Function Hoisting in Javascript
Function declarations are hoisted along with their entire body, allowing you to call functions before their actual declaration in the code.
hello();
Output: "Hello, World!"
function hello() {
console.log("Hello, World!");
}
6. Block Scoping and Hoisting
Hoisting also occurs within block scopes, but the variables are hoisted to the top of their enclosing function, not the block.
function test() {
console.log(a); // Output: undefined
var a = 30;
if (true) {
var b = 40;
}
console.log(b); // Output: 40
}
test();
7. Benefits of Hoisting in Javascript
Hoisting can lead to cleaner code and improve readability by allowing you to declare variables and functions at the top of their scope.
8. Hoisting Pitfalls
Although hoisting can be beneficial, it can also lead to unexpected behavior if not understood properly. It’s essential to be aware of potential pitfalls.
9. Hoisting Best Practices
To leverage hoisting effectively, follow these best practices:
- Always declare variables at the top of their scope.
- We explicitly initialize variables and declare functions where they are needed instead of relying on hoisting for clarity.
By understanding hoisting and its implications, you can unlock its full potential and write more robust and maintainable JavaScript code.
The below references cover various aspects of Hoisting in JavaScript and provide in-depth explanations and examples for further understanding. For more information, visit: Hoisting in JavaScript