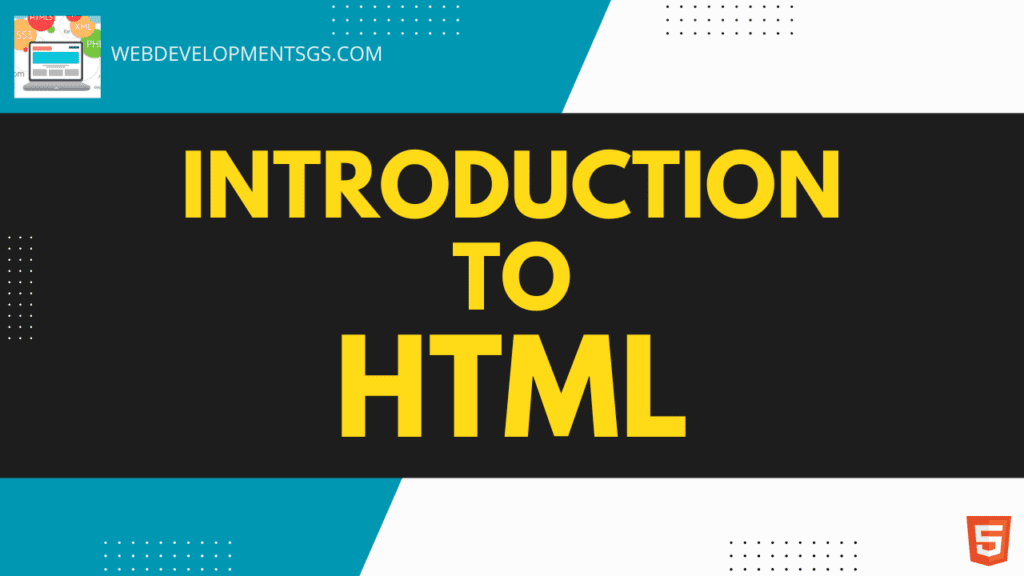
HTML, or HyperText Markup Language, is the fundamental basis of web development. This language is both user-friendly and powerful, allowing for the effective structuring and presentation of online content. HTML is essential for anyone looking to dive into the world of website creation, as it forms the backbone of almost every webpage online. Whether you’re a curious beginner or a budding web developer, understanding HTML basics is crucial for creating websites that are both functional and visually appealing.
In this comprehensive guide, we’ll explore HTML basics, including its structure, essential elements, and how to build your first webpage. This HTML tutorial covers everything you need to know to get started with web development, breaking down complex concepts into simple steps.
What is HTML?
HTML, or HyperText Markup Language, serves as a markup language designed to organize content on the internet. It establishes the structure of web pages by arranging text, images, links, and various other components in a format that is easy to read and suitable for the web.
Unlike programming languages that focus on logic and computations, HTML is primarily concerned with the presentation of content. For example, <h1> tags define headers, <p> tags define paragraphs, and <a> tags define links. HTML tags are surrounded by angle brackets (e.g., <tag>), and they come in pairs: an opening tag and a closing tag, the latter marked with a forward slash (e.g., </tag>).
Why HTML is Important in Web Development
HTML forms the basic structure of all websites. This is why it is crucial in the field of web development:
- Foundation of Web Pages: HTML is the core framework that underpins all websites. Without it, a browser would not know how to display or organize content. Learn more: MDN Web Docs – HTML Basics
- Cross-Browser Compatibility: HTML is standardized across all web browsers, ensuring that content appears similarly on Chrome, Firefox, Safari, and others. Explore: Can I Use – Browser Support Tables for HTML Features
- Easy to Learn: HTML is beginner-friendly and requires minimal setup. It’s often the first language taught in web development courses.
- SEO Relevance: Proper use of HTML tags can improve a webpage’s search engine visibility. Search engines like Google rely on HTML tags to understand page content.
Understanding HTML basics is the first step in becoming proficient in web development. Once you grasp HTML basics, adding styles with CSS and functionality with JavaScript becomes much easier.
HTML Basics: Key Concepts and Structure
Before we dive into the code, let’s review some essential HTML concepts:
1. HTML Document Structure
Every HTML document follows a standard structure, containing specific sections to organize the content. Here’s a breakdown of a basic HTML document:
<!DOCTYPE html>
<html>
<head>
<title>My First Webpage</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>Welcome to my webpage.</p>
</body>
</html>
Explanation:
- <!DOCTYPE html>: Declares the document type as HTML5, the latest HTML version.
- <html>: The root element of the HTML document.
- <head>: Contains meta-information about the document, like the title, character set, and links to stylesheets.
- <title>: Assigns the page title that can be seen in the tab of the browser.
- <body>: Contains all the content displayed on the page, like text, images, and links.
2. Tags and Elements
HTML relies on tags to define content types. Tags are defined by angle brackets, which include both an initial opening tag and a final closing tag. For example, <p> represents a paragraph element, and the content appears between <p> and </p>.
Learn more: MDN Web Docs – HTML Elements Reference
Common Tags:
- <h1> to <h6>: Header tags define section headings, with <h1> as the highest level and <h6> the lowest.
- <p>: Defines a paragraph.
- <a>: Defines a hyperlink.
- <img>: Embeds an image (self-closing).
- <ul> and <ol>: used to create different type of unordered and ordered lists.
- <li>: Represents list items within <ul> or <ol>.
3. Attributes
HTML tags frequently include attributes that offer supplementary details regarding an element. Attributes are written within the opening tag, and each attribute has a name and value.
For example:
<a href="https://www.example.com" target="_blank">Visit Example</a>
In this example:
- href specifies the URL the link points to.
- target=”_blank” makes the link open in a new tab.
Building Blocks of HTML: A Step-by-Step Tutorial
Let’s put theory into practice by building a simple webpage.To establish a basic webpage through HTML, please follow these outlined steps:
Step 1: Setting Up the HTML Document
Start a text editor like Notepad or Visual Studio Code and create a new document. Remember to save this document with the .html extension, such as index.html.
Download Visual Studio Code: Official Site
Step 2: Adding the Document Structure
Start with the basic structure mentioned earlier. Copy and paste the following code into your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>My Web Development Journey</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is my first webpage using HTML!</p>
</body>
</html>
Step 3: Adding More Elements
Let’s add more content to make our webpage informative and visually engaging.
1. Adding a Link
<p>Learn more about <a href="https://www.htmlbasics.com">HTML basics</a>.</p>
More tutorials: W3Schools HTML Tutorial
2. Adding an Image
Use the <img> tag to add an image. The src attribute specifies the path to the image, and alt provides alternative text for accessibility.
<img src="path/to/image.jpg" alt="A descriptive image" width="300">
Step 4: Organizing Content with Headings and Lists
Headings and lists are essential for structuring content.
<h2>Benefits of Learning HTML</h2>
<ul>
<li>Easy to learn and use</li>
<li>Foundation of web development</li>
<li>Improves SEO</li>
</ul>
HTML Best Practices for Beginners
The following are important practices to follow for effective HTML writing:
- Keep Code Clean and Indented: Organize your code with consistent indentation. This improves readability, especially for larger projects.
- Use Semantic Tags: HTML5 introduced semantic tags like <header>, <footer>, <article>, and <section>, which enhance accessibility and SEO.
- Close All Tags: Although some HTML tags can be self-closing, always close non-self-closing tags to avoid errors.
- Add Comments for Clarity: Adding (<!– comment –>) comments to your code makes it more comprehensible, especially in the context of teamwork.
Advanced HTML Elements for Enhanced Web Development
After mastering the basics, you can explore advanced HTML elements to create more interactive and dynamic web content.
1. Forms
Forms are designed to collect user data through input fields like text boxes, radio buttons, and buttons for submission.
<form action="/submit_form" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
2. Tables
Tables display data in rows and columns, using <table>, <tr> (table row), <th> (table header), and <td> (table data) tags.
<table>
<tr>
<th>Name</th>
<th>Age</th>
</tr>
<tr>
<td>John</td>
<td>25</td>
</tr>
</table>
3. Multimedia Elements
HTML provides the capability to embed audio and video content seamlessly.
Audio:
<audio controls>
<source src="path/to/audio.mp3" type="audio/mpeg">
</audio>
Video:
<video width="320" height="240" controls>
<source src="path/to/video.mp4" type="video/mp4">
</video>
Common HTML Errors and Troubleshooting Tips
- Unclosed Tags: Forgetting to close tags can cause layout issues.
- Incorrect Nesting: Elements should be arranged in a manner that ensures they are properly nested.
- Case Sensitivity: While HTML tags are case-insensitive, it’s best practice to use lowercase for consistency.
- Broken Links/Paths: Double-check file paths and URLs to ensure they’re correct.
Conclusion
Mastering the HTML basics, is a crucial foundational step in the journey of web development. This guide has provided a foundation in HTML, from structuring a webpage to using essential tags, attributes, and advanced elements. With consistent practice, you’ll gain the confidence to create more complex websites and add interactivity through CSS and JavaScript.
For further exploration:
For beginners, mastering HTML can open doors to various opportunities in web design, development, and digital marketing. Start building, experimenting, and soon you’ll be creating dynamic and engaging websites!